In Osclass v8.2.0, we brought possibility to use multiple category-based hooks for item publish and item edit pages. Until now, there was one and only one hook for publish/edit, that was very limiting. This hook was usually shown at the end of publish/edit page. (Keep in mind default one still need to exists on publish/edit pages!).
Predefined variants (if your theme has these integrated already):
- item_form_top
- item_form_category
- item_form_description
- item_form_price
- item_form_location
- item_form_seller
- item_form_images
- item_form_hook
- item_form_buttons
- item_form_bottom
- item_form_after
- item_edit_top
- item_edit_category
- item_edit_description
- item_edit_price
- item_edit_location
- item_edit_seller
- item_edit_images
- item_edit_hook
- item_edit_buttons
- item_edit_bottom
- item_edit_after
To use predefined variant, you just hook your function to it.
Warning! Not meant to use all of these. Each variant generates 1 ajax call.
Function for publish page:
ItemForm::plugin_post_item();
Function for edit page:
ItemForm::plugin_edit_item();
Hook and parameter variants
In new version, we added optional parameters to these 2 functions:
- $hook_variant – customize hook and use “item_form_{$variant}” and “item_edit_{$variant}” hook instead of default one
- $param_variant – pass custom parameter value to item_form & item_edit hooks. Parameter name is “variant”.
Hook variant
Let’s say you want to create new category-based hook and show data right after category selection. You do not want to impact current functions in any way.
1) Create custom function that shows some data (does not need to be category related):
function my_custom_func($catId) { echo '<div style="padding:15px 15px 15px 30%;background:#ccc;display:inline-block;width:100%;margin:0 0 10px 0;">'; echo 'Category ID is: ' . $catId; echo '</div>'; }
2) Think about unique variant to use and hook this function to item publish and edit page. Let’s use variant “vcategory”. You will hook new function using these commands:
osc_add_hook('item_form_vcategory', 'my_custom_func'); osc_add_hook('item_edit_vcategory', 'my_custom_func');
3) Update your theme item-post.php (maybe item-edit.php is required as well!). We consider theme use just item-post.php. You want to show new data below category selection.
a) Place following code below category box (note ie on sigma theme this would be around line 70, not needed if theme has variants integrated already and you want to use existing one):
<?php if(Params::getParam('action') == 'item_edit') { ItemForm::plugin_edit_item('vcategory'); } else { ItemForm::plugin_post_item('vcategory'); } ?>
b) Alternative way is to use new design hooks (if supported by your theme) and show box using hook:
osc_add_hook('item_publish_category', function() { if(Params::getParam('action') == 'item_edit') { ItemForm::plugin_edit_item('vcategory'); } else { ItemForm::plugin_post_item('vcategory'); } });
Summary – whole code you can place ie. to theme functions.php:
function my_custom_func($catId) { echo '<div style="padding:15px 15px 15px 30%;background:#ccc;display:inline-block;width:100%;margin:0 0 10px 0;">'; echo 'Category ID is: ' . $catId; echo '</div>'; } osc_add_hook('item_form_vcategory', 'my_custom_func'); osc_add_hook('item_edit_vcategory', 'my_custom_func'); osc_add_hook('item_publish_category', function() { if(Params::getParam('action') == 'item_edit') { ItemForm::plugin_edit_item('vcategory'); } else { ItemForm::plugin_post_item('vcategory'); } });
Now you should see something like this (sigma):
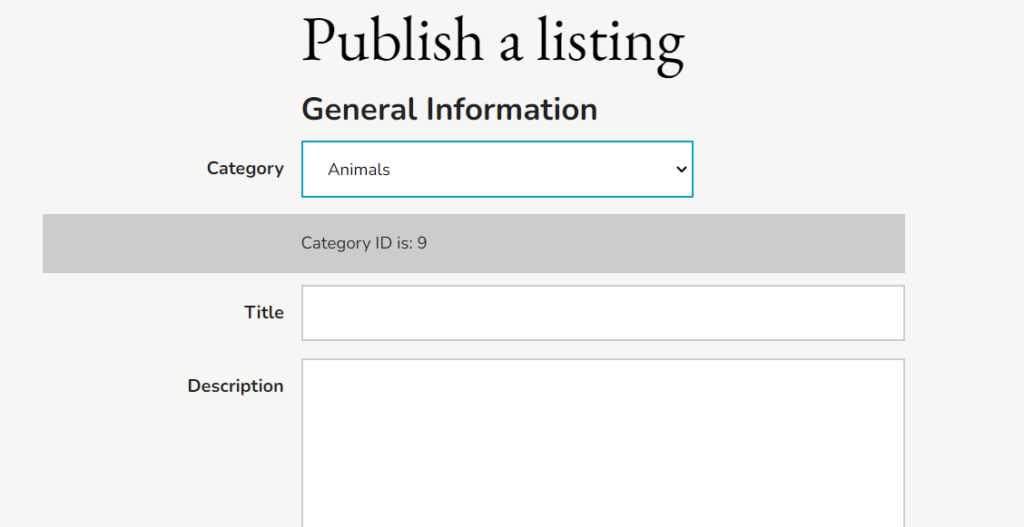
Note: if your theme has these hooks integrated, code related to calling ItemForm functions is not required at all! Do not add it, otherwise you will get duplicated results.
Parameter variants
Parameter variants allows to parametrize existing function hooked to item_form and item_edit. In this case no new hooks are used, but parameter “variant” is available.
Params::getParam('variant')
It’s equal to empty string if no parameter variant is provided.
In this case, you need to place new hooks in following form:
if(Params::getParam('action') == 'item_edit') { ItemForm::plugin_edit_item('', 'pcategory'); } else { ItemForm::plugin_post_item('', 'pcategory'); }
Note that you cannot use parameter variants and hook variants at same time. Hook variant will be prioritized and parameter variant ignored.